
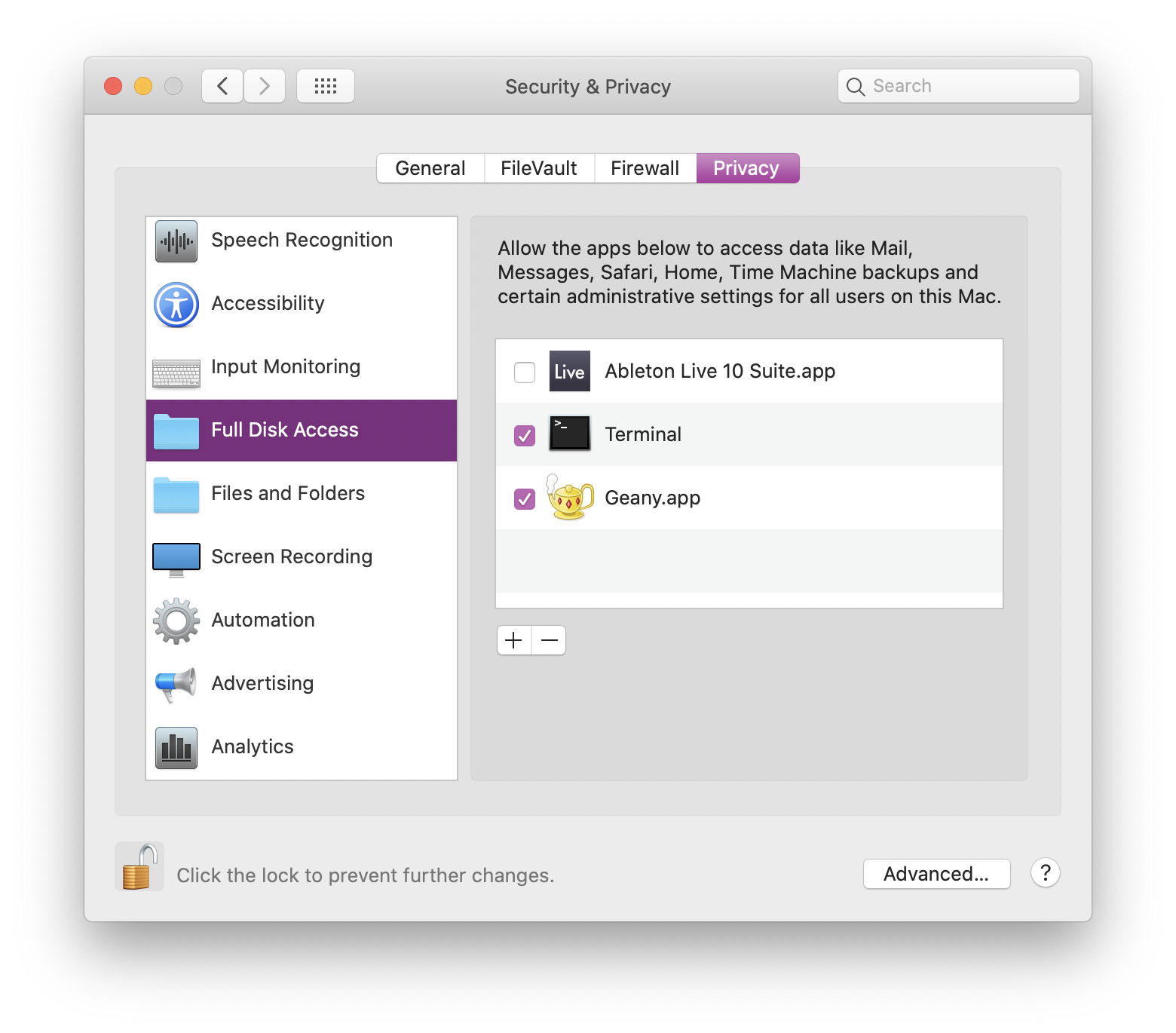
Run() − The run() method is the entry point for a thread. The methods provided by the Thread class are as follows − In addition to the methods, the threading module has the Thread class that implements threading. Threading.enumerate() − Returns a list of all thread objects that are currently active. Threading.currentThread() − Returns the number of thread objects in the caller's thread control. Threading.activeCount() − Returns the number of thread objects that are active. The threading module exposes all the methods of the thread module and provides some additional methods − The newer threading module included with Python 2.4 provides much more powerful, high-level support for threads than the thread module discussed in the previous section. You will have to press ctrl-c to stopĪlthough it is very effective for low-level threading, the thread module is very limited compared to the newer threading module.
PYTHON LOCK FILE FOR ACCESS ON MAC CODE
When the above code is executed, it produces the following result − _thread.start_new_thread( print_time, ("Thread-2", 4, ) ) _thread.start_new_thread( print_time, ("Thread-1", 2, ) ) Print ("%s: %s" % ( threadName, time.ctime(time.time()) ))

kwargs is an optional dictionary of keyword arguments. Here, args is a tuple of arguments use an empty tuple to call function without passing any arguments. When the function returns, the thread terminates. The method call returns immediately and the child thread starts and calls function with the passed list of args. This method call enables a fast and efficient way to create new threads in both Linux and Windows. _thread.start_new_thread ( function, args ) To spawn another thread, you need to call the following method available in the thread module − However, it has been renamed to "_thread" for backwards compatibilities in Python3. Hence, in Python 3, the module "thread" is not available anymore. Users are encouraged to use the threading module instead. The thread module has been "deprecated" for quite a long time. There are two modules which support the usage of threads in Python3 − Kernel Threads are a part of the operating system, while the User-space threads are not implemented in the kernel. There are two different kind of threads − It can temporarily be put on hold (also known as sleeping) while other threads are running - this is called yielding. It has an instruction pointer that keeps track of where within its context is it currently running. Threads are sometimes called light-weight processes and they do not require much memory overhead they are cheaper than processes.Ī thread has a beginning, an execution sequence, and a conclusion. Multiple threads within a process share the same data space with the main thread and can therefore share information or communicate with each other more easily than if they were separate processes. All potentially competing processes must use the "AtomicOpen" class.Running several threads is similar to running several different programs concurrently, but with the following benefits − WARNING: The locking provided here is advisory, not absolute.
PYTHON LOCK FILE FOR ACCESS ON MAC WINDOWS
WARNING: If running on Windows and Python crashes before exit is called, I'm not sure what the lock behavior would be. Now, "AtomicOpen" could be used wherever one would normally use an "open" statement. # default any exceptions are raised to the user # Handle exceptions that may have come up during execution, by # Unlock the file and close the file objectĭef _exit_(self, exc_type=None, exc_value=None, traceback=None): # the user did not have the AtomicOpen in a "with" block. # Allows users to use the 'close' function if they want, in case # Return the opened file object (knowing a lock has been obtained)ĭef _enter_(self, *args, **kwargs): return self.file # Open the file and acquire a lock on the file before operating # a lock on that file object (WARNING: Advisory locking)ĭef _init_(self, path, *args, **kwargs): # Open the file with arguments provided by user. # initialization like a standard call to 'open' that happens to be atomic # Class for ensuring that all file operations are atomic, treat Msvcrt.locking(f.fileno(), msvcrt.LK_UNLCK, file_size(f)) Msvcrt.locking(f.fileno(), msvcrt.LK_RLCK, file_size(f))

Return os.path.getsize( os.path.realpath(f.name) ) # Posix based file locking (Linux, Ubuntu, MacOS, etc.) If you would prefer to do it yourself, here is some code for a cross-platform solution that uses the respective file locking tools on Linux / DOS systems.
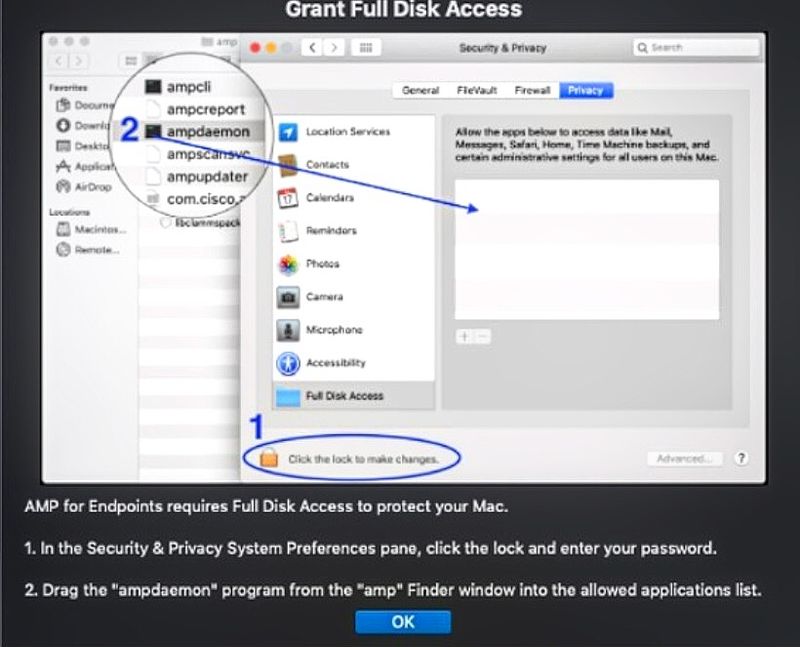
The other solutions cite a lot of external code bases.
